How to Add Structured Data to Blog Posts in Gatsby
Adding structured data to your blog posts with react-helmet is a simple way to display previews of your content
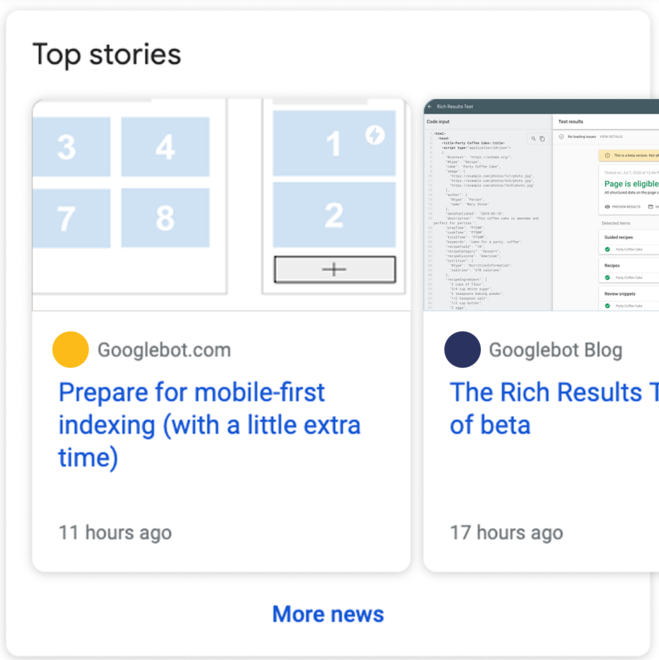
Structured data tells search engines the exact content of a webpage, and is complementary to meta tags.
Structured data is important for SEO because it makes it easier for Google to understand what your blog post and website are about. Google will use your structured data to show a rich search result, which are always displayed at the top of results, putting your website in the best position for increased click through rates.
Using React Helmet
React Helmet is the best way to add structured data with Gatsby. Start by installing it via npm
npm install gatsby-plugin-react-helmet react-helmet
and add it to your plugin array inside of gatsby-config.js
plugins: [
gatsby-plugin-react-helmet]
We'll create a separate component called StructuredData.js
where we initialize react-helmet. We'll pass in an article
prop so that we show previews for blog posts, but not for other pages on our sites (which would cause errors).
StructuredData.js
import React from "react"
import { Helmet } from "react-helmet"
const StructuredData = ({ article }) => {
return (
<Helmet title="Structured Data Example">
{(article ? true : null) && (
<script type="application/ld+json">
{JSON.stringify({
"@context": "https://schema.org",
"@type": "BlogPosting",
mainEntityOfPage: {
"@type": "WebPage",
"@id": `https://codesandbox.io/s/competent-darkness-7m3h7?file=/src/StructuredData.js`
},
headline: `How to Add Structured Data to Blog Posts in Gatsby`,
description: `Adding structured data to your blog posts with react-helmet is a simple way to display previews of your content`,
image: `https://developers.google.com/search/docs/data-types/images/amp-article.png`,
author: {
"@type": "Organization",
name: `Snappy Web Design`
},
publisher: {
"@type": "Organization",
name: `Snappy Web Design`,
logo: {
"@type": "ImageObject",
url:
"https://images.ctfassets.net/z7pyyvho21dm/56G3Sy04rYABwiRJE0Qpd5/93c58230cd554417689228cab8a4f077/Screenshot_2021-04-26_132720.png?w=680&h=144&q=50&fm=webp"
}
},
datePublished: `2021-06-07`,
dateModified: `2021-06-07`
})}
</script>
)}
</Helmet>
)
}
export default StructuredData
Then we import the component inside of BlogPost.js
to add structured data to our blog posts:
BlogPost.js
import React from "react"
import StructuredData from "../StructuredData"
const BlogPost = () => (
<>
<StructuredData article={true} />
<h1>This is my blog post</h1>
<p>Welcome to my blog post with structured data</p>
<p>To the top of the google search results we come!</p>
</>
)
export default BlogPost
Wrapup - You're done!
Use the Rich Results Test provided by Google to make sure you've set everything up correctly and check your data is coming through as expected.
If everything looks good - you're done! Adding structured data to your blog posts in gatbsy with react-helmet is as easy as 1-2-3! You can check out the final code here
Make sure to press the follow button below to be notified when new posts go live! 🔔
More from Snappy Web Design
Subscribe to the Snappy Web Design Blog to get notified when a new post goes live. We'll never share your e-mail address or use it for any reason other than to share new posts.
Published May 5
Discover the secrets to designing a website that speaks to your small business's unique identity
Published September 1
Snappy Web Design is the one-stop-shop for web design.
Published August 0
A horrifying trip through poor UX design